We’re all told to have strong passwords for everything. Long, good, complicated passwords with symbols, numbers, capital letters. Passwords that are a pain to enter without a passwords manager, in essence. Because password managers exist, that’s all good for nearly every situation, except when it comes to logging in to your desktop.
I know this kind of thing exists already, but I don’t think they exist quite as simply (and potentially as un-securely) as this.
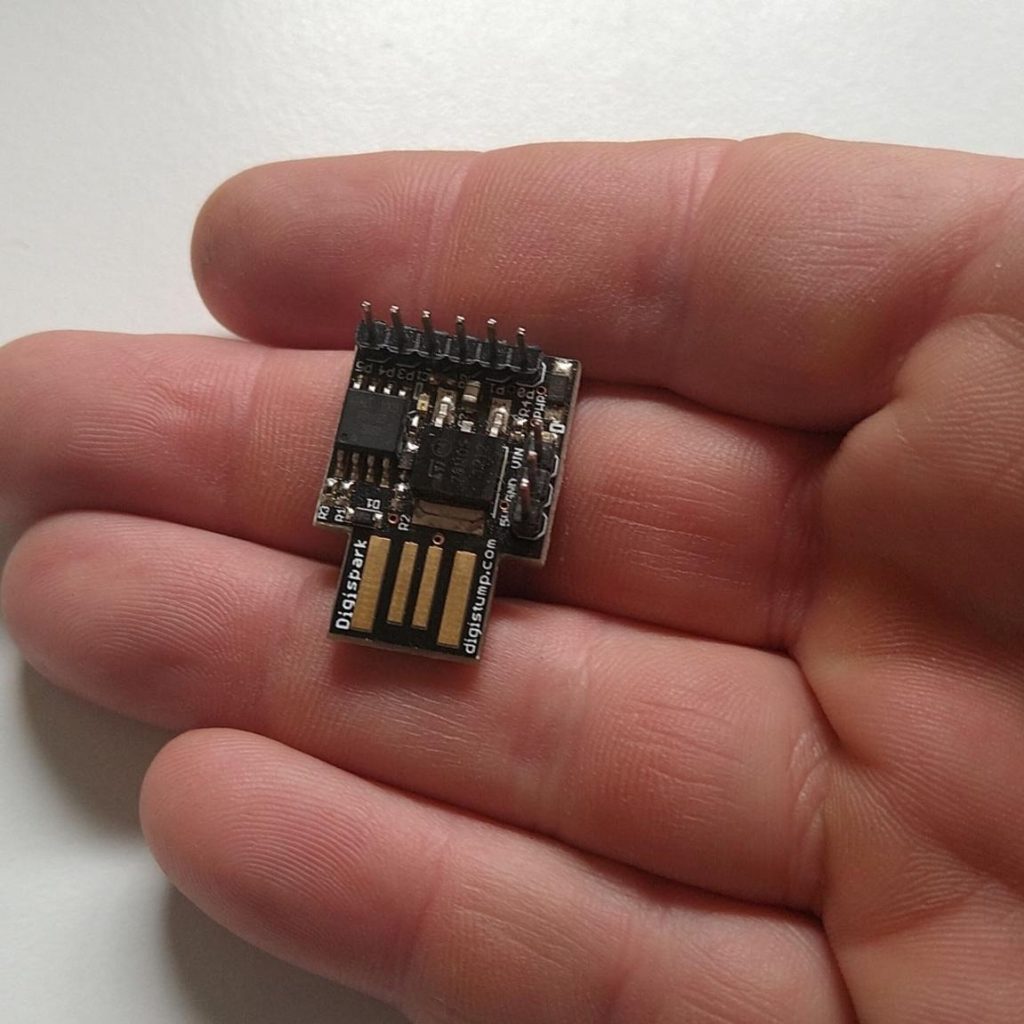
What I’ve made, using an old Digispark ATTiny85 arduino board with a built in USB-A plug, is a device that, when plugged in to a USB port, and the button is pressed, will type in a pre-determined string of characters followed by the enter key. There are two use-cases I can think of for this. Logging in to your desktop where there’s no password manager available, and entering your long-winded password into your password manager when setting that up.
There are obviously security issues with this – it’s a single factor authentication system. And you’re keeping the entire password available to anyone who picks up the doodad, plugs it in, and presses the button. There are things that could be done to make that safer – like only keeping part of the long-winded password on the device, requiring user input for to complete the password. But as a little one-hour project, this was fun.
My soldering is a mess, but it works. The button circuit includes a pull-up resistor, to stop any erratic button behaviour when there isn’t a definite high or low signal, as per a tonne of tutorials online. Like this, straight from the Arduino source: https://www.arduino.cc/en/Tutorial/BuiltInExamples/Button
Here’s the completed product:
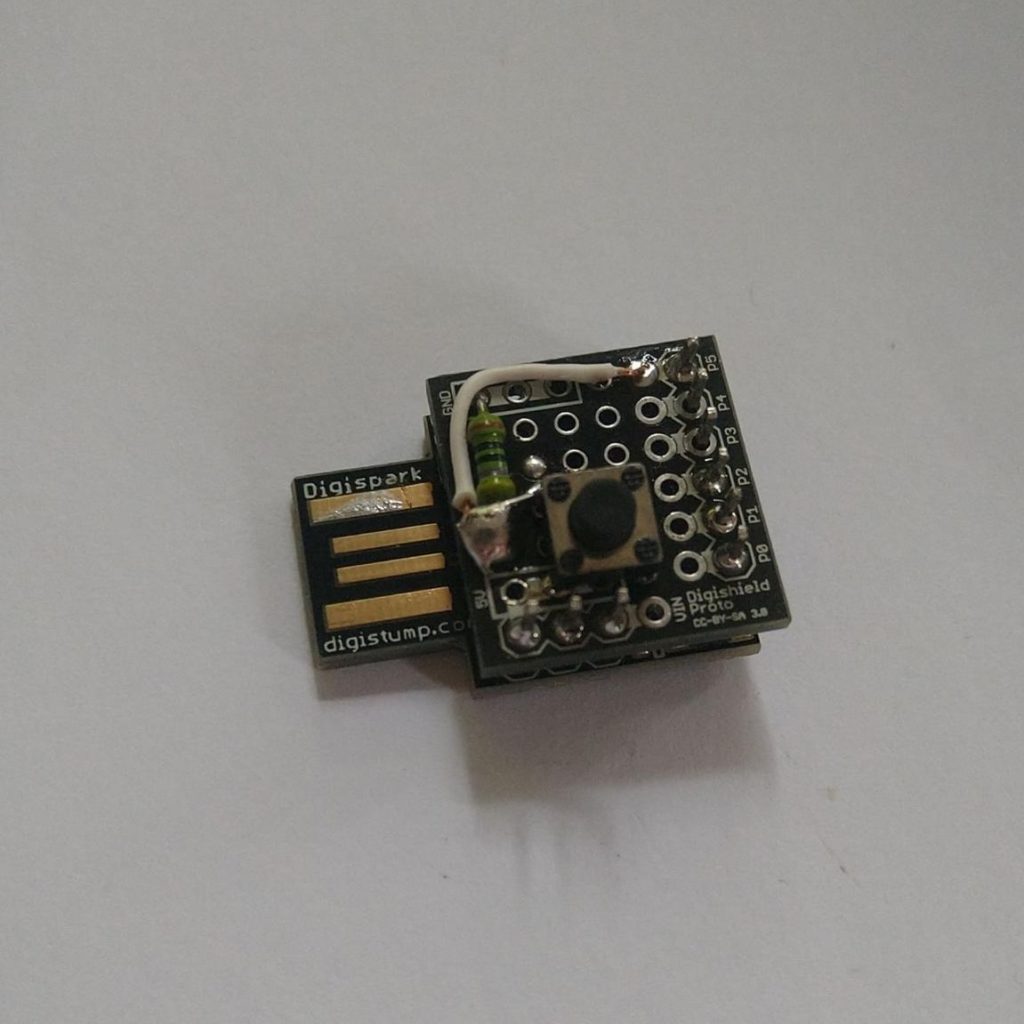
The code that’s running it is as below. Firstly, import the HID library from Digispark (there are tutorials on how to get that happening on the Digispark website). Initialise the button state, configure the required pin to wait for signal, then repeatedly wait for button press. If there’s a button press, send the defined string to the computer, and to prevent multiple entries, wait for a second before moving on.
#include "DigiKeyboard.h"
int buttonState = 0;
void setup() {
pinMode(5, INPUT);
}
void loop() {
buttonState = digitalRead(5);
if (buttonState == HIGH) {
DigiKeyboard.sendKeyStroke(0);
DigiKeyboard.println("notmyactualpassword");
DigiKeyboard.delay(1000);
}
}
You could add a fingerprint reader?
Getting a bit advanced!